Assignment 1
Assignment 2
Assignment 1 : Simplified rendering pipeline
Update 2/03: Color interpolation across vertices is now part of the
regular assignment, and the extra credit has been changed to clipping.
There is a flaw in the image result for test 6, the correct version is
here.
Details below.
Overview
In this assignment, you will be implementing a simplified 3D rendering
pipeline (with flat shading). This will consist of several parts:
1. vertex and viewing transformations
2. rasterization
3. using a z-buffer for hidden surfaces
Code
Skeleton code is available HERE.
Submission Instructions
Deadline February 7th.
Your assignment is to implement the functions in
minigl.cpp. To submit your assignment, submit it on ilearn
1. your implementation of minigl.cpp
2. a file called documentation.txt that gives a very brief
explanation of how you implemented each function, as well as any part of
the assignment that isn't working properly.
Submit on iLearn by 5pm 2/7.
Software Framebuffer
You will have a software framebuffer that stores both a 2D array of pixel colors and a 2D array of z (depth) values.
Your rasterization routine will write into this software framebuffer.
Rasterization and z-buffer depth test
Implement a routine that draws a filled triangle specified by three
vertices into your software framebuffer. The routine should write its
result to the software framebuffer, doing the appropriate z-buffer
check.
Vertex and viewing transformations
Similar to OpenGL, you will maintain both a projection matrix stack and a
modelview stack.
When the user draws a vertex you will apply the modelview and projection
( projection * (modelview * vertex) ) to obtain
the transformed geometry. This is followed by a divide by w, and a
viewport transform. You will then store this transformed geometry for
rasterization. The reason for this is that
the transformations are part of the current state and will not
necessarily persist.
Getting Started
If you need help getting started, I suggest you do the following:
- Get the mglBegin(), mglEnd(), and mglVertex3() calls working first. Draw a wireframe of the triangles using the line algorithm you implemented in lab. mglVertex3() call should add vertices to the vertexList. While mglEnd() should iterate through that list and rasterize each element.
- from http://www.songho.ca
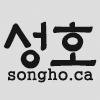
- Make sure your transformations are working before you implement mglPushMatrix() and mglPopMatrix().
- You can still get a bulk of your grade, even if you can only rasterize your polygons in wireframe
- Each function that you are expected to write in miniGL corresponds
to an appropriate OpenGL command. I strongly recommend you look at THIS before implementing the appropriate functions.
Extra Credit
Extra credit can be earned by implementing geometric clipping. Test this by taking one of the existing tests and modifying it so that part of the image falls outside the
viewing volume. Geometric clipping means modifying polygon(s) that fall outside the volume to stay within it, not going through pixel by pixel and removing them if they're
outside the array bounds. Mention how you did this and tested it in your documentation.
Grading Breakdown
70 pts : Transformations
10 pts : Matrix operations (such as mglMultMatrix)
30 pts : Modelview transformations (scale, rotate, translate)
30 pts : Projection
10 pts : Polygon rasterization
10 pts : Polygon color interpolation
10 pts : Depth buffer
10 pts : (extra credit) Implement geometric clipping
Note: this is subject to change.
Assignment 2 : Ray Tracing
Description
In this assignment, you will implement a basic ray tracer. The images above depict the 4 test images you code will be
able to produce. The images depict flat shading, phong shading, addition of shadows, and addition of reflections.
Code
Skeleton code is available HERE.
There are 4 files:
1. main.cpp: You do not need to modify this file.
2. ray_tracer.h: This contains the declarations for the classes and methods you will need to implement.
3. ray_tracer.cpp: This is where you should write your implementation.
4. Makefile
The methods that you need to implement are marked with 'TODO' in the code. A code skeleton and driver framework is
provided. You will need to implement object intersections, and shading, and the ray casting.
To run the tests, you run
./ray_tracer < test number >
where test number is 1-4.
You can implement the tests in order:
Test 1: You will need to set up the basic infrastructure for this test, but the flat shader will suffice. You
also do not need to do any shadow or reflection rays for this test.
Test 2: Here you will add the Phong Shader. The phong shading will depend on the lighting, so you will need
lights.
Test 3: In this test you will generate shadows by casting shadow rays.
Test 4: Here you will make your ray tracer recursive. You will implement the Reflective_Shader and cast
reflection rays.
Submission Instructions
1. your implementation of 'ray_tracer.cpp'
2. a file called 'documentation.txt' that gives a very brief
explanation of how you implemented each function and what was or was not
working.
Extra Credit
There are several options for extra credit:
1. Implement texture mapping. E.g., map a checkboard texture onto the ground plane.
2. Implement environment mapping. Use a background image for the environment and render a scene where we can see the
reflection of the background image in a reflective surface, or
3. Implement a transparent shader.